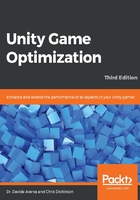
Faster GameObject null reference checks
It turns out that performing a null reference check against a GameObject will result in some unnecessary performance overhead. GameObjects and MonoBehaviours are special objects compared to a typical C# object, in that they have two representations in memory: one exists within the memory managed by the same system managing the C# code we write (managed code), whereas the other exists in a different memory space, which is handled separately (native code). Data can move between these two memory spaces, but each time this happens will result in some additional CPU overhead and possibly an extra memory allocation.
This effect is commonly referred to as crossing the Native-Managed Bridge. If this happens, it is likely to generate an additional memory allocation for an object's data to get copied across the bridge, which will require the garbage collector to eventually perform some automatic cleanup of memory for us. This subject will be explored in much more detail in Chapter 8, Masterful Memory Management, but for the time being, just consider that there are many subtle ways to accidentally trigger this extra overhead, and a simple null reference check against GameObject is one of them:
if (gameObject != null) {
// do stuff with gameObject
}
An alternative that generates a functionally equivalent output that operates around twice as quickly (although it does obfuscate the purpose of the code a little) is System.Object.ReferenceEquals():
if (!System.Object.ReferenceEquals(gameObject, null)) {
// do stuff with gameObject
}
This applies to both GameObjects and MonoBehaviours, as well as other Unity objects, which have both native and managed representations such as the WWW class. However, some rudimentary testing reveals that either null reference check approach still consumes mere nanoseconds on an Intel Core i5 3570K processor. So, unless you are performing massive amounts of null reference checks, the gains might be marginal at best. However, this is a warning worth keeping in mind for the future, as it will come up a lot.