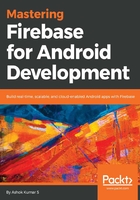
Facebook login
The Facebook configuration that we carried out in the FirebaseUI method is very similar, but here we will have to do all the customization according the application. We need the app id and and app protocol scheme ID. Before all of this, we need to add a valid hash key to the Facebook developer console. Once we have done all this configuration, we have to add the meta tag to the manifest:
<meta-data android:name="com.facebook.sdk.ApplicationId"
android:value="@string/facebook_application_id"/>
Add the Firebase dependency to your app's Gradle file.
implementation 'com.facebook.android:facebook-android-sdk:[4,5)'
After the library is synced with the project, we will have access to the following button class, which has the button designed for facebook login:
<com.facebook.login.widget.LoginButton
android:text="Button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/loginButton"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintTop_toBottomOf="@+id/statusText" />
Now, after this, register a callbackManager in the oncreate method:
callbackManager = CallbackManager.Factory.create();
And set the required parameter permissions:
loginButton.setReadPermissions("email", "public_profile");
Now, we can add these callbacks to the loginButton:
loginButton.registerCallback(callbackManager,
new FacebookCallback<LoginResult>() {
@Override
public void onSuccess(LoginResult loginResult) {
Log.d(TAG, "onSuccess: " + loginResult);
}
@Override
public void onCancel() {
Log.d(TAG, "onCancel: User cancelled sign-in");
}
@Override
public void onError(FacebookException error) {
Log.d(TAG, "onError: " + error);
}
});
Now, we need to override the onActivityResult method:
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
callbackManager.onActivityResult(requestCode, resultCode, data);
}
We have successfully integrated Facebook with the application; now, we need to register and exchange tokens, and that can be achieved as shown here:
private void registerToFirebase(AccessToken token) {
AuthCredential credential =
FacebookAuthProvider.getCredential(token.getToken());
FirebaseAuth.signInWithCredential(credential)
.addOnCompleteListener(this, new OnCompleteListener<AuthResult>() {
@Override
public void onComplete(@NonNull Task<AuthResult> task) {
if (!task.isSuccessful()) {
Toast.makeText(FacebookAuthActivity.this,
"Authentication failed.",
Toast.LENGTH_SHORT).show();
}
}
});
}
This explains how to integrate Facebook and Firebase together without using any UI frameworks.