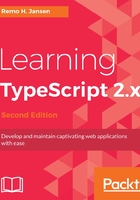
上QQ阅读APP看书,第一时间看更新
Namespaces
Namespaces, also known as internal modules, are used to encapsulate features and objects that share a certain relationship. Namespaces will help you to organize your code. To declare a namespace in TypeScript, you will use the namespace and export keywords:
In older versions of TypeScript, the keyword to define an internal module was module instead of namespace.
namespace geometry { interface VectorInterface { /* ... */ } export interface Vector2DInterface { /* ... */ } export interface Vector3DInterface { /* ... */ } export class Vector2D implements VectorInterface, Vector2dInterface { /* ... */ } export class Vector3D implements VectorInterface, Vector3DInterface { /* ... */ } } let vector2DInstance: geometry.Vector2DInterface = new geometry.Vector2D(); let vector3DInstance: geometry.Vector3DInterface = new geometry.Vector3d();
In the preceding code snippet, we have declared a namespace that contains the classes vector2D and vector3D and the interfaces VectorInterface, Vector2DInterface, and Vector3DInterface. Note that the first interface is missing the keyword export. As a result, the interface VectorInterface will not be accessible from outside the module's scope.
Namespaces are a good way to organize your code; however, they are not the recommended way to organize your code in a TypeScript application. We will not get into more details about this topic for now, but we will learn more about internal and external modules and we'll discuss when each is appropriate and how to use them in Chapter 4 , Object-Oriented Programming with TypeScript.