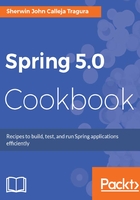
How to do it...
To implement page redirection with Flash-scoped objects, apply these steps:
- To start our experiment on implementing page redirection, let us create a controller that loads a login form, processes the username and password, and then redirects to another form controller through Spring's old way of page navigation, that is, through RedirectView:
@Controller public class RedirectPageController { @RequestMapping(value="/login.html", method=RequestMethod.GET) public String login(){ return "login"; } @RequestMapping(value="/jump_page.html", method=RequestMethod.POST) public RedirectView sendRedirection(RedirectAttributes atts, @RequestParam("username") String username, @RequestParam("password") String password){ atts.addFlashAttribute("username", username); atts.addFlashAttribute("password", password); atts.addAttribute("request", "loginForm"); return new RedirectView("/redirectviewOld.html",true); } @RequestMapping(value="/redirectviewOld.html", method=RequestMethod.GET) public String resultPageOld(Model model ){ return "result_page"; } }
The handler method uses org.springframework.web.servlet.mvc.support.RedirectAttributes to convert username and password parameters to Flash-scoped objects. Flash-scoped objects are capable of holding their values until the next redirected view is processed. Likewise, they can also pass typical request-scoped objects to success views.
- Create the login form view with typical HTML textboxes and submit buttons:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <%@ taglib prefix="spring" uri="http://www.springframework.org/tags" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html;charset=ISO-8859-1"> <title><spring:message code="login_title" /></title> </head> <body> <form action="/ch03/jump_page.html" method="POST"> <spring:message code="userLbl" /> <input type="text" name="username" /><br/> <spring:message code="passwordLbl" /> <input type="text" name="password" /><br/> <input type="submit" value="Login" /> </form> </body> </html>
- Create the redirected page that will show on screen both the username and password Flash-scoped objects and a request-scoped one:
<%@ page language="java" contentType="text/html;charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>Insert title here</title> </head> <body> User Name: ${ username } <br/> Password: ${ password } <br/> Requested by: ${ loginForm } </body> </html>
- Update the views.properties and messages_en_US.properties for all the updates on the views and the view's message bundles.
- Save all files and deploy the project. Open a browser and execute https://localhost:8443/ch03/login.html. Observe how the two Flash-scoped and a request-scoped data behave when in this form:

- This submits to another form controller using page redirection:
- Add the following request handlers that use a modern technique of implementing page redirection through the shorthand keyword redirect:
@RequestMapping(value="/new_jump.html", method=RequestMethod.GET) public ModelAndView sendRedirectionModel(ModelMap atts){ atts.addAttribute("pageId_flash", "12345"); return new ModelAndView( "redirect:/redirectviewNew.html",atts); } @RequestMapping(value="/redirectviewNew.html", method=RequestMethod.GET) public String resultPageNew(Model model, @ModelAttribute("pageId_flash") String flash){ model.addAttribute("pageId_flash", flash); return "new_result_page"; }
There are three conditions expected to be met when using the prefix redirect, and one is the absence of RedirectAttributes for Flash-scoped object generation. Also, this technique is best paired with ModelAndView with regard to the transporting of objects to views. And lastly, the redirected handler method must have @ModelAttribute to fetch all objects passed by the originator request from which the redirection started.
- Save all files. Then clean, build, and deploy the project. Run https://localhost:8443/ch03 /new_jump.html on a browser and expect this output:
